You can listen to user inputs using various methods in JavaScript. Some of the most popular ones are given here with examples :
Example 1
The value keyword can be used to capture the user input inside the DOM. Here’s how it works :
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
Enter Name : <input type="text" id="name" />
<button id="btn">Display Name</button>
<p>Click button to see output inside console</p>
</body>
<script src="index.js"></script>
</html>
document.getElementById("btn").onclick = function () {
let nameDisplay = document.getElementById("name").value;
console.log(nameDisplay);
};
Inside the JavaScript code block above you’ll see that we’ve used a keyword called onclick (used on button) which runs a function to display the name to the console using the value keyword.
Here’s how it looks :
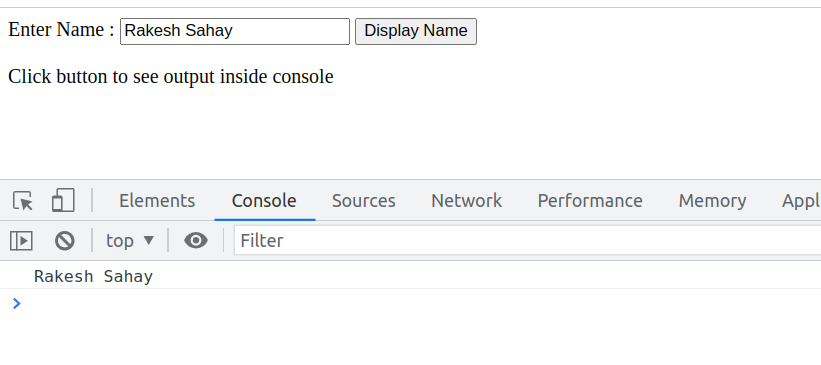
Example 2
In this example, rather then logging the text to the console we’re typing the text just beneath the input field. Here’s how :
<body>
<input type="text" placeholder="your text here" id="text" />
<p id="typed-text"></p>
<script src="index.js"></script>
</body>
We have a paragraph field (p) here and the below JS code displays the user input between the <p> and </p> tags.
const inputText = document.querySelector("input");
const log = document.getElementById("typed-text");
inputText.addEventListener("input", function textEntry(anyThing) {
log.textContent = anyThing.target.value;
});
In the above code block we’ve used the target.value keyword to display the user input in real-time. Unlike in Example 1 there’s no ‘click’ event here.
Here’s how it shows up :
